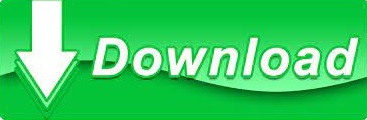
If you just want to tweak some minor parts of the website, it might be overkill to include big libraries which will slow down the site.īesides, frameworks and libraries come and go. But some web pages are just static with very little logic. Well, yeah, you are probably right, most of the time. Doesn’t modern web application use frameworks and libraries like Angular, ReactJS or VueJS? You might be wondering what is the point of creating this in vanilla JavaScript.

This file must be in the same directory as your index.html file for this to work. Remember to include the people.json file as well. As an exercise, you can try to style the output to look nicer. Try to copy and paste this in your own editor. Var mainContainer = document.getElementById("myData") We can then get every object in our list of JSON object and append it into our main div. Step 2 – Loop through every object in our JSON object This is vanilla JavaScript and this is how we did front-end development in the “old” days :). This will find the element with the id myData. We get the element by executing the getElementByID function. This is how we will do it: var mainContainer = document.getElementById("myData") We need this because we are going to fill the div with the people in our JSON file. Remember the div with the myData id from our index.html ? We want to fetch that div using JavaScript. Step 1 – Get the div element from the body
JSON DATA CREATOR LOCAL MACHINE FULL
Our goal is to just simply display the full name of the people in our JSON file. However, we will do this in a simple and ugly way. We could create a table and make it look really good with nice styling. There are several ways to display the data in our HTML. Our plan is to fill our JSON data inside this div dynamically. Pretty simple right? We have just a simple div with the id myData. Displaying the JSON dataīefore we display our JSON data on the webpage, let’s just see how the body of our index.html file looks like. Normally you would display an error message to the user if something went wrong. Notice that in our catch function, we are just writing the error message to out console.
JSON DATA CREATOR LOCAL MACHINE CODE
This is where we create the code which will append the data to our page. Notice that we are calling a function called appendData. Now we can take this data and display it on our HTML page. This data looks just like the data in our JSON file. In the second then function we get the actual JSON data as a parameter. This is why we just return it and chain another then function.

The json() function also returns a promise. To get the JSON data from the response, we execute the json() function. After the file has been read from disk, we run the then function with the response as a parameter. Here we are fetching our people.json file. Let us see how this will look in out example: fetch('people.json') If anything goes wrong (like the JSON file cannot be found), the catch function will run. When the JSON data is fetched from the file, the then function will run with the JSON data in the response. The fetch function will return a promise.

We don’t have to drill down to any directory since the json file is in the same directory as our index.html. In our case it is just the filename people.json.
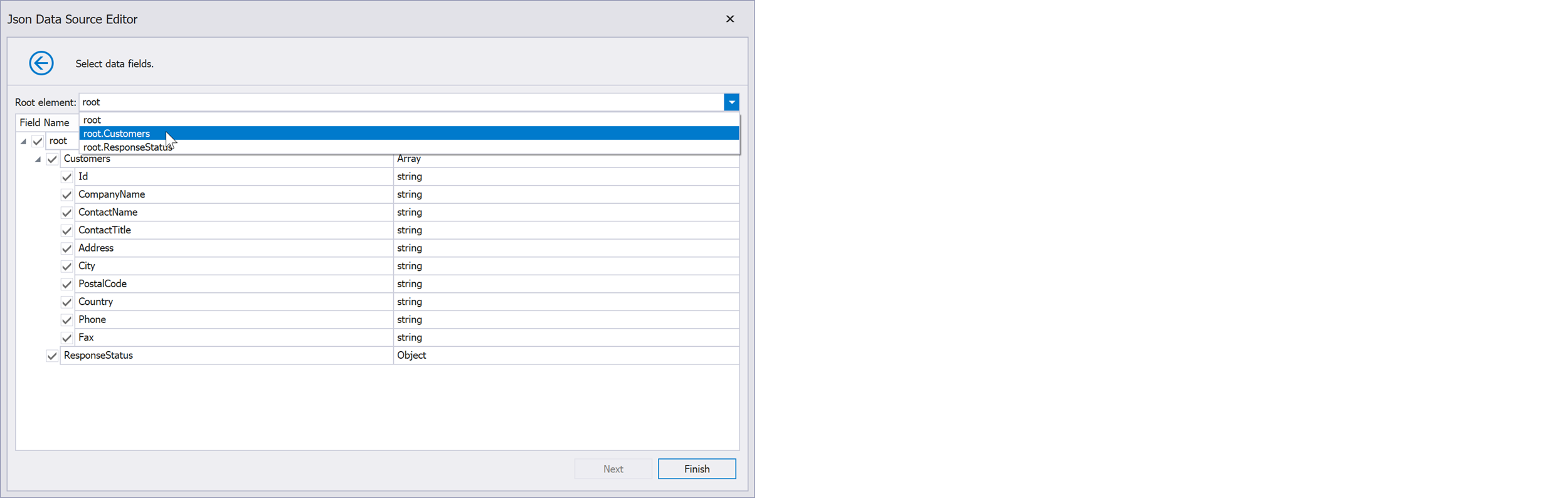
The url parameter used in the fetch function is where we get the JSON data. If an error occured, you will catch it here We use the fetch API in the following way: fetch(url) We will fetch this data by using the fetch API. To be able to display this data in our HTML file, we first need to fetch the data with JavaScript. 3 Why use Vanilla JavaScript? Fetching the JSON data
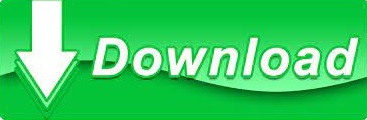